Table of Contents
Vercel has recently been adding a feature called the third-party library, which allows us to integrate various third-party tools like Google Analytics in the most performant way possible without writing much code or using the Nextjs script tag.
@next/third-parties is a library that provides a collection of components and utilities that improve the performance and developer experience of loading popular third-party libraries in your Next.js application.
Installing @next/third-parties
As it’s currently in the development phase it’s recommended to use it with the latest version of Nextjs or Nextjs Canary.
Use the below command to install @next/third-parties and the latest version of Nextjs
npm install @next/third-parties@latest next@latest
If you are already working in the latest version of Nextjs just run this command
npm install @next/third-parties@latest
Google Third-Parties
Although all supported third-party libraries from Google can be imported from @next/third-parties/google
we are going to use only Google Analytics
Google Analytics
The GoogleAnalytics
component can be used to include Google Analytics 4 on your page.
To load Google Analytics for all routes, include the component directly in your root layout and pass in your measurement ID:
import { GoogleAnalytics } from '@next/third-parties/google'
export default function RootLayout({
children,
}: {
children: React.ReactNode
}) {
return (
<html lang="en">
<body>{children}</body>
<GoogleAnalytics gaId="G-XYZ" />
</html>
)
}
Here gaid
is the Google Analytics 4 ID which you can find in your Google Analytics dashboard, use this guide from google to find your gaid
.
To load Google Analytics for a single route, include the component in your page file:
import { GoogleAnalytics } from '@next/third-parties/google'
export default function Page() {
return <GoogleAnalytics gaId="G-XYZ" />
}
Row run your application with ‘npm run dev` and use it in the browser for few seconds and you might see realtime update in Google Analytics
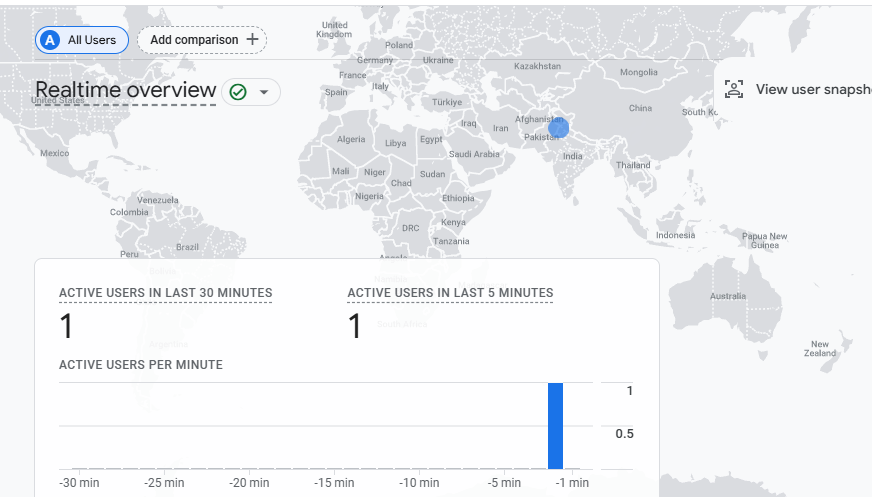
Sending events
The sendGAEvent
function can be used to measure user interactions on your page by sending events using the dataLayer
object. For this function to work, the <GoogleAnalytics />
component must be included in either a parent layout, page, or component, or directly in the same file.
'use client'
import { sendGAEvent } from '@next/third-parties/google'
export function EventButton() {
return (
<div>
<button
onClick={() => sendGAEvent('event', 'buttonClicked', { value: 'xyz' })}
>
Send Event
</button>
</div>
)
}
For more details visit official guide.